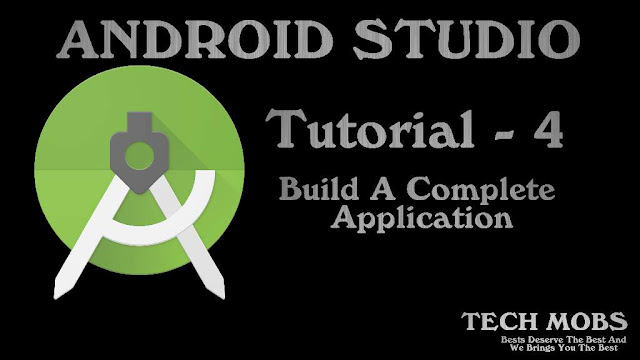
Watch the video and follow me :
- Build a new Android Studio Project .
- I am using the package name as com.techmobs.app here you can change it to any other name that you like , but be care full while copy pasting the codes don't make the package name on each files wrong be sure that you use the same package name every where .
- Choose the Navigation Drawer Activity .
- After gradle build up we can move on to the AndroidManifest.xml file
- Open AndroidManifest.xml file and select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Now move on to the MainActivity.java file .
- Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Open Projects .
- Download the volley.jar file , copy and paste it in the libs folder .
- Left click on the volley.jar file and select Add as Library option and click ok . Now the gradle will build and after that you need to go back to Android .
- Create a new java class with name UpdateDialog .
- Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Create another java class with name UpdateService .
- Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Now we need to add an assets folder , left click on the app , then select new , then select folder , then select Assets Folder and click Finish .
- Now create a file in the assets , left click then select new then File .
- Name the file update.html , paste the following codes in the file .
- We need to add a new activity , again at app left click and select new then select Activity then Empty Activity .
- Name the activity as splash , then the layout name automatically becomes activity_splash .
- Now we will get to files splash.java and activity_splash.xml .
- Open splash.java file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Open res , layout , activity_splash.xml . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Now the activity_main.xml file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Open app_bar_main.xml file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Open content_main.xml file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Open nav_header_main.xml file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Open the res , menu folder , activity_main_drawer.xml file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Open main.xml file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Now open the folder res , values , colors.xml file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Open styles.xml file . Select the entire codes and replace it with the code bellow . Don't make package name error , be careful .
- Now go through the java parts again to change the links , name , email , etc
- First of all the MainActivity.java file , open it and replace the website urls with your website url . Watch video for more clarification .
- Like that the splash.java file replace the facebook , google+ , twitter links .
- Now the UpdateService.java "https://vishnutechmobs.github.io/update-link.html" this is the link link which is checked when the application opens . The content in line
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<manifest xmlns:android="http://schemas.android.com/apk/res/android" | |
package="com.techmobs.app"> | |
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" /> | |
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> | |
<uses-permission android:name="android.permission.READ_LOGS" /> | |
<uses-permission android:name="android.permission.KILL_BACKGROUND_PROCESSES" /> | |
<uses-permission android:name="android.permission.INTERNET" /> | |
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> | |
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" /> | |
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" /> | |
<uses-permission android:name="android.permission.CHANGE_WIFI_STATE" /> | |
<uses-permission android:name="android.permission.CHANGE_NETWORK_STATE"/> | |
<application | |
android:allowBackup="true" | |
android:icon="@mipmap/ic_launcher" | |
android:label="@string/app_name" | |
android:supportsRtl="true" | |
android:theme="@style/AppTheme"> | |
<activity | |
android:name=".MainActivity" | |
android:label="@string/app_name" | |
android:theme="@style/AppTheme.NoActionBar"> | |
<intent-filter> | |
<action android:name="android.intent.action.MAIN" /> | |
<category android:name="android.intent.category.DEFAULT" /> | |
</intent-filter> | |
</activity> | |
<activity | |
android:name=".splash" | |
android:label="@string/app_name"> | |
<intent-filter> | |
<action android:name="android.intent.action.MAIN" /> | |
<category android:name="android.intent.category.LAUNCHER" /> | |
</intent-filter> | |
</activity> | |
<activity android:name=".UpdateDialog" | |
android:theme="@style/Animation.AppCompat.Dialog"/> | |
<service | |
android:name=".UpdateService" | |
android:enabled="true" | |
android:exported="true"/> | |
</application> | |
</manifest> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package com.techmobs.app; | |
import android.content.Context; | |
import android.content.DialogInterface; | |
import android.content.Intent; | |
import android.graphics.Bitmap; | |
import android.net.ConnectivityManager; | |
import android.net.NetworkInfo; | |
import android.net.Uri; | |
import android.os.AsyncTask; | |
import android.os.Bundle; | |
import android.support.annotation.NonNull; | |
import android.support.design.widget.FloatingActionButton; | |
import android.support.v7.app.AlertDialog; | |
import android.view.KeyEvent; | |
import android.view.View; | |
import android.support.design.widget.NavigationView; | |
import android.support.v4.view.GravityCompat; | |
import android.support.v4.widget.DrawerLayout; | |
import android.support.v7.app.ActionBarDrawerToggle; | |
import android.support.v7.app.AppCompatActivity; | |
import android.support.v7.widget.Toolbar; | |
import android.view.Menu; | |
import android.view.MenuItem; | |
import android.webkit.WebSettings; | |
import android.webkit.WebView; | |
import android.webkit.WebViewClient; | |
import android.widget.ImageView; | |
import android.widget.ProgressBar; | |
import android.widget.TextView; | |
import android.widget.Toast; | |
import org.json.JSONObject; | |
import java.io.BufferedReader; | |
import java.io.InputStreamReader; | |
import java.net.URL; | |
public class MainActivity extends AppCompatActivity | |
implements NavigationView.OnNavigationItemSelectedListener { | |
private String ver = "1.0"; | |
private WebView wv; | |
@Override | |
protected void onCreate(Bundle savedInstanceState) { | |
super.onCreate(savedInstanceState); | |
setContentView(R.layout.activity_main); | |
/*----------- Update activity start --------------*/ | |
Intent intent = new Intent(MainActivity.this, UpdateService.class); | |
startService(intent); | |
/*----------- Update activity end -----------------*/ | |
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar); | |
toolbar.setTitle("TECH MOBS"); | |
setSupportActionBar(toolbar); | |
final ProgressBar progressBar = (ProgressBar) findViewById(R.id.prb); | |
wv = (WebView) this.findViewById(R.id.webview); | |
wv.setWebViewClient(new WebViewClient() { | |
@Override | |
public void onPageStarted(WebView view, String url, Bitmap favicon) { | |
super.onPageStarted(view, url, favicon); | |
progressBar.setVisibility(View.VISIBLE); | |
} | |
@Override | |
public boolean shouldOverrideUrlLoading(WebView webView, String url) | |
{ | |
if(url.contains("tipsandtricksonandroid.blogspot")) { | |
webView.loadUrl(url); | |
} else startActivity(new Intent("android.intent.action.VIEW", Uri.parse(url))); | |
return true; | |
} | |
@Override | |
public void onPageFinished(WebView view, String url) { | |
super.onPageFinished(view, url); | |
progressBar.setVisibility(View.GONE); | |
} | |
}); | |
WebSettings webSettings = wv.getSettings(); | |
webSettings.setJavaScriptEnabled(true); | |
webSettings.getAllowContentAccess(); | |
webSettings.getAllowFileAccess(); | |
webSettings.setDatabaseEnabled(true); | |
webSettings.setAppCacheEnabled(true); | |
if(isInternetAvailable()) { | |
wv.loadUrl("https://tipsandtricksonandroid.blogspot.com/"); | |
} else { | |
progressBar.setVisibility(View.GONE); | |
wv.setVisibility(View.GONE); | |
TextView tv = (TextView) findViewById(R.id.nointv); | |
tv.setVisibility(View.VISIBLE); | |
} | |
FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab); | |
fab.setOnClickListener(new View.OnClickListener() { | |
@Override | |
public void onClick(View view) { | |
final Intent intent2 = new Intent("android.intent.action.SEND"); | |
intent2.setType("message/rfc822"); | |
intent2.putExtra("android.intent.extra.EMAIL", new String[]{"techmobs.in@gmail.com"}); | |
intent2.putExtra("android.intent.extra.SUBJECT", "TECH MOBS v" + ver); | |
intent2.putExtra("android.intent.extra.TEXT", ""); | |
try { | |
startActivity(Intent.createChooser(intent2, "Report...")); | |
} catch (Exception ex) { | |
Toast.makeText(MainActivity.this, "Can't find email client.", Toast.LENGTH_SHORT).show(); | |
} | |
} | |
}); | |
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout); | |
ActionBarDrawerToggle toggle = new ActionBarDrawerToggle( | |
this, drawer, toolbar, R.string.navigation_drawer_open, R.string.navigation_drawer_close); | |
drawer.setDrawerListener(toggle); | |
toggle.syncState(); | |
NavigationView navigationView = (NavigationView) findViewById(R.id.nav_view); | |
navigationView.setNavigationItemSelectedListener(this); | |
setupNavigationHeader(navigationView); | |
} | |
@Override | |
public void onBackPressed() { | |
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout); | |
if (drawer.isDrawerOpen(GravityCompat.START)) { | |
drawer.closeDrawer(GravityCompat.START); | |
} else { | |
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(MainActivity.this); | |
alertDialogBuilder.setTitle("Exit"); | |
alertDialogBuilder | |
.setMessage("Do you really want to exit?") | |
.setCancelable(false) | |
.setPositiveButton("Yes",new DialogInterface.OnClickListener() { | |
public void onClick(DialogInterface dialog,int id) { | |
MainActivity.this.finish(); | |
} | |
}) | |
.setNegativeButton("No",new DialogInterface.OnClickListener() { | |
public void onClick(DialogInterface dialog,int id) { | |
dialog.cancel(); | |
} | |
}).show(); | |
} | |
} | |
@Override | |
public boolean onCreateOptionsMenu(Menu menu) { | |
getMenuInflater().inflate(R.menu.main, menu); | |
return true; | |
} | |
@Override | |
public boolean onOptionsItemSelected(MenuItem item) { | |
int id = item.getItemId(); | |
if (id == R.id.action_whatsapp) { | |
startActivity(new Intent("android.intent.action.VIEW", Uri.parse("https://chat.whatsapp.com/6NuAILVAIVjEjP6MaC0rCR"))); | |
} else if (id == R.id.action_fb) { | |
startActivity(new Intent("android.intent.action.VIEW", Uri.parse("https://www.facebook.com/tipsandtricksonandroid/"))); | |
} | |
else if (id == R.id.action_gplus) { | |
startActivity(new Intent("android.intent.action.VIEW", Uri.parse("https://plus.google.com/+VISHNUS%20TRICKS"))); | |
} | |
return super.onOptionsItemSelected(item); | |
} | |
@SuppressWarnings("StatementWithEmptyBody") | |
@Override | |
public boolean onNavigationItemSelected(@NonNull MenuItem item) { | |
int id = item.getItemId(); | |
if (id == R.id.nav_youtube) { | |
wv.loadUrl("https://www.youtube.com/c/vishnusivadas"); | |
} else if (id == R.id.notice) { | |
this.wv.loadUrl("https://vishnutechmobs.github.io/notice.html"); | |
}else if (id == R.id.nav_exit) { | |
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(this); | |
alertDialogBuilder.setTitle("Exit"); | |
alertDialogBuilder.setMessage("Do you really want to exit?") .setCancelable(false) | |
.setPositiveButton("Yes",new DialogInterface.OnClickListener() { | |
public void onClick(DialogInterface dialog,int id) { | |
MainActivity.this.finish(); | |
} | |
}) | |
.setNegativeButton("No",new DialogInterface.OnClickListener() { | |
public void onClick(DialogInterface dialog,int id) { | |
dialog.cancel(); | |
} | |
}).show(); | |
} | |
else if (id == R.id.nav_home) { | |
this.wv.loadUrl("https://tipsandtricksonandroid.blogspot.in/"); | |
}else if (id == R.id.nav_blog) { | |
this.wv.loadUrl("https://tipsandtricksonandroid.blogspot.in/search?max-result=10"); | |
}else if (id == R.id.nav_about) { | |
this.wv.loadUrl("https://vishnutechmobs.github.io/about.html"); | |
} else if (id == R.id.nav_contact) { | |
this.wv.loadUrl("http://bit.do/contact-techmobs"); | |
} else if (id == R.id.nav_share) { | |
final String string = "Download Our Application At : https://tipsandtricksonandroid.blogspot.in/p/techmob-application.html Share It To All And Enjoy ....:)"; | |
final Intent intent3 = new Intent("android.intent.action.SEND"); | |
intent3.setType("text/plain"); | |
intent3.putExtra("android.intent.extra.SUBJECT", "TECH MOBS"); | |
intent3.putExtra("android.intent.extra.TEXT", string); | |
startActivity(Intent.createChooser(intent3, "Share")); | |
} | |
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout); | |
drawer.closeDrawer(GravityCompat.START); | |
return true; | |
} | |
@Override | |
public boolean onKeyDown(int keyCode, KeyEvent event) | |
{ | |
if((keyCode == KeyEvent.KEYCODE_BACK)&& wv.canGoBack()) | |
{ | |
wv.goBack(); | |
return true; | |
} | |
return super.onKeyDown(keyCode, event); | |
} | |
//other | |
private boolean isInternetAvailable() { | |
ConnectivityManager connectivityManager | |
= (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE); | |
NetworkInfo activeNetworkInfo = connectivityManager.getActiveNetworkInfo(); | |
return activeNetworkInfo != null && activeNetworkInfo.isConnected(); | |
} | |
public void setupNavigationHeader(NavigationView mNavigationView) { | |
View headerView = mNavigationView.getHeaderView(0); | |
TextView navAppVersion = (TextView) headerView.findViewById(R.id.tv); | |
try { | |
navAppVersion.setText("techmobs.in@gmail.com"); | |
} catch (Exception e) { | |
e.printStackTrace(); | |
} | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package com.techmobs.app; | |
import android.app.Activity; | |
import android.app.AlertDialog; | |
import android.content.DialogInterface; | |
import android.content.Intent; | |
import android.net.Uri; | |
import android.os.Bundle; | |
import android.webkit.WebView; | |
import android.webkit.WebViewClient; | |
import android.widget.Toast; | |
// Copyright owner vishnu | |
// Official website : www.tipsandtricksonandroid.blogspot.com | |
// Created on : 26-april-2017 // | |
public class UpdateDialog extends Activity { | |
@Override | |
protected void onCreate(Bundle savedInstanceState) { | |
super.onCreate(savedInstanceState); | |
final AlertDialog alertDialog = new AlertDialog.Builder(this).create(); | |
alertDialog.setTitle("Update Available"); | |
WebView wv = new WebView(this); | |
wv.loadUrl("file:///android_asset/update.html"); | |
wv.setWebViewClient(new WebViewClient() { | |
@Override | |
public boolean shouldOverrideUrlLoading(WebView view, String url) { | |
view.loadUrl(url); | |
return true; | |
} | |
}); | |
alertDialog.setView(wv); | |
alertDialog.setButton(DialogInterface.BUTTON_POSITIVE, "Download Now", new DialogInterface.OnClickListener() { | |
@Override | |
public void onClick(DialogInterface dialog, int which) { | |
Intent intent = new Intent(Intent.ACTION_VIEW); | |
intent.setData(Uri.parse("https://vishnutechmobs.github.io/update.html")); | |
if (intent.resolveActivity(getPackageManager()) != null) { | |
startActivity(intent); | |
Toast.makeText(UpdateDialog.this, "Download And Enjoy", Toast.LENGTH_LONG).show(); | |
} | |
} | |
}); | |
alertDialog.setButton(DialogInterface.BUTTON_NEGATIVE, "Update Later", new DialogInterface.OnClickListener() { | |
public void onClick(DialogInterface dialog, int which) { | |
finish(); | |
Toast.makeText(UpdateDialog.this, "Don't forget to update", Toast.LENGTH_LONG).show(); | |
} | |
}); | |
alertDialog.show(); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package com.techmobs.app; | |
import android.app.Service; | |
import android.content.Intent; | |
import android.os.IBinder; | |
import android.widget.Toast; | |
import com.android.volley.Request; | |
import com.android.volley.RequestQueue; | |
import com.android.volley.Response; | |
import com.android.volley.VolleyError; | |
import com.android.volley.toolbox.StringRequest; | |
import com.android.volley.toolbox.Volley; | |
// Copyright owner vishnu | |
// Official website : www.tipsandtricksonandroid.blogspot.com | |
// Created on : 26-april-2017 // | |
public class UpdateService extends Service { | |
public UpdateService() { | |
} | |
@Override | |
public int onStartCommand(Intent intent, int flags, int startId) { | |
RequestQueue queue = Volley.newRequestQueue(getApplicationContext()); | |
String url = "https://vishnutechmobs.github.io/update-link.html"; | |
StringRequest stringRequest = new StringRequest(Request.Method.GET, url, new Response.Listener<String>() { | |
@Override | |
public void onResponse(String response) { | |
if (response != null) { | |
boolean resp = response.contains("1.0"); | |
if (!resp) { | |
//New Update Available | |
Intent intent1 = new Intent(UpdateService.this,UpdateDialog.class); | |
intent1.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); | |
startActivity(intent1); | |
} else { | |
Toast.makeText(UpdateService.this, "All Good", Toast.LENGTH_LONG).show(); | |
} | |
} | |
} | |
}, new Response.ErrorListener() { | |
@Override | |
public void onErrorResponse(VolleyError volleyError) { | |
volleyError.printStackTrace(); | |
} | |
}); | |
queue.add(stringRequest); | |
return Service.START_NOT_STICKY; | |
} | |
@Override | |
public void onDestroy() { | |
super.onDestroy(); | |
} | |
@Override | |
public IBinder onBind(Intent intent) { | |
// TODO: Return the communication channel to the service. | |
throw new UnsupportedOperationException("Not yet implemented"); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!DOCTYPE html> | |
<html> | |
<head> | |
<style type='text/css'> | |
div.title2 | |
{ | |
color: #025F53; | |
font-size: 1.5em; | |
margin-top: 1em; | |
text-align: center; | |
font-family: 'Niconne', cursive; | |
} | |
.normal | |
{ | |
text-align: center; | |
} | |
</style> | |
<body style="background-color:transparent;"> | |
<marquee behavior="alternate" scrollamount="10"><h1>Update Available</h1></marquee> | |
<div class='title2' > <marquee scrollamount="3" width="25">***</marquee> | |
Developed By Vishnu | |
<marquee scrollamount="3" width="25" direction="right">***</marquee></div> | |
<br/><br/> | |
<div class='normal'>A new version of this application is out</div><br/><br/> | |
</body> | |
</html> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package com.techmobs.app; | |
import android.content.Intent; | |
import android.graphics.Typeface; | |
import android.net.Uri; | |
import android.os.Handler; | |
import android.support.v7.app.AppCompatActivity; | |
import android.os.Bundle; | |
import android.view.View; | |
import android.widget.TextView; | |
public class splash extends AppCompatActivity { | |
@Override | |
protected void onCreate(Bundle savedInstanceState) { | |
super.onCreate(savedInstanceState); | |
setContentView(R.layout.activity_splash); | |
Handler handler = new Handler(); | |
handler.postDelayed(new Runnable() { | |
@Override | |
public void run() { | |
startActivity(new Intent(splash.this, MainActivity.class)); | |
finish(); | |
} | |
},3000); | |
} | |
public void browser1(View view){ | |
Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse("https://www.facebook.com/tipsandtricksonandroid")); | |
startActivity(browserIntent); | |
} | |
public void browser2(View view){ | |
Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse("https://plus.google.com/+VISHNUS%20TRICKS")); | |
startActivity(browserIntent); | |
} | |
public void browser3(View view){ | |
Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse("https://twitter.com/vishnusiva123")); | |
startActivity(browserIntent); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<RelativeLayout | |
xmlns:android="http://schemas.android.com/apk/res/android" | |
xmlns:tools="http://schemas.android.com/tools" | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" | |
tools:context="com.techmobs.app.splash" | |
android:background="@drawable/splash_img" | |
tools:layout_editor_absoluteY="25dp" | |
tools:layout_editor_absoluteX="0dp"> | |
<TextView | |
android:id="@+id/textView3" | |
android:layout_width="154dp" | |
android:gravity="center" | |
android:layout_height="39dp" | |
android:text="Loading..." | |
android:textColor="@color/colorWhite" | |
android:textSize="20sp" | |
android:textStyle="italic" | |
tools:layout_editor_absoluteX="115dp" | |
tools:layout_editor_absoluteY="92dp" | |
android:layout_above="@+id/progressBar3" | |
android:layout_centerHorizontal="true" /> | |
<ProgressBar | |
android:id="@+id/progressBar3" | |
style="?android:attr/progressBarStyle" | |
android:layout_width="wrap_content" | |
android:layout_height="wrap_content" | |
android:foregroundGravity="bottom|center" | |
tools:layout_editor_absoluteX="168dp" | |
tools:layout_editor_absoluteY="4dp" | |
android:layout_marginBottom="28dp" | |
android:layout_alignParentBottom="true" | |
android:layout_alignRight="@+id/googlesplash" | |
android:layout_alignEnd="@+id/googlesplash" /> | |
<Button | |
android:id="@+id/googlesplash" | |
android:layout_width="50dp" | |
android:layout_height="50dp" | |
android:background="@drawable/gplus" | |
android:clickable="true" | |
android:onClick="browser2" | |
android:shape="ring" | |
android:layout_alignTop="@+id/facebooksplash" | |
android:layout_centerHorizontal="true" /> | |
<Button | |
android:id="@+id/facebooksplash" | |
android:layout_width="50dp" | |
android:layout_height="50dp" | |
android:layout_alignParentLeft="true" | |
android:layout_alignParentStart="true" | |
android:layout_alignTop="@+id/twittersplash" | |
android:layout_marginLeft="13dp" | |
android:layout_marginStart="13dp" | |
android:background="@drawable/fb" | |
android:clickable="true" | |
android:onClick="browser1" | |
android:shape="ring" /> | |
<Button | |
android:id="@+id/twittersplash" | |
android:layout_width="42dp" | |
android:layout_height="42dp" | |
android:layout_alignParentEnd="true" | |
android:layout_alignParentRight="true" | |
android:layout_alignParentTop="true" | |
android:layout_marginEnd="13dp" | |
android:layout_marginRight="13dp" | |
android:layout_marginTop="50dp" | |
android:background="@drawable/twitter" | |
android:clickable="true" | |
android:onClick="browser3" | |
android:shape="ring" /> | |
</RelativeLayout> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<android.support.v4.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android" | |
xmlns:app="http://schemas.android.com/apk/res-auto" | |
xmlns:tools="http://schemas.android.com/tools" | |
xmlns:fab="http://schemas.android.com/apk/res-auto" | |
android:id="@+id/drawer_layout" | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" | |
android:fitsSystemWindows="true" | |
tools:openDrawer="start"> | |
<include | |
layout="@layout/app_bar_main" | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" /> | |
<android.support.design.widget.NavigationView | |
android:id="@+id/nav_view" | |
android:layout_width="wrap_content" | |
android:layout_height="match_parent" | |
android:layout_gravity="start" | |
android:fitsSystemWindows="true" | |
app:headerLayout="@layout/nav_header_main" | |
app:menu="@menu/activity_main_drawer"> | |
</android.support.design.widget.NavigationView> | |
</android.support.v4.widget.DrawerLayout> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" | |
xmlns:app="http://schemas.android.com/apk/res-auto" | |
xmlns:tools="http://schemas.android.com/tools" | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" | |
android:fitsSystemWindows="true" | |
tools:context="com.techmobs.app.MainActivity"> | |
<android.support.design.widget.AppBarLayout | |
android:layout_width="match_parent" | |
android:layout_height="wrap_content" | |
android:theme="@style/AppTheme.AppBarOverlay"> | |
<android.support.v7.widget.Toolbar | |
android:id="@+id/toolbar" | |
android:layout_width="match_parent" | |
android:layout_height="?attr/actionBarSize" | |
android:background="?attr/colorPrimary" | |
app:popupTheme="@style/AppTheme.PopupOverlay" /> | |
</android.support.design.widget.AppBarLayout> | |
<include | |
android:id="@+id/tv" | |
layout="@layout/content_main" /> | |
<android.support.design.widget.FloatingActionButton | |
android:id="@+id/fab" | |
android:layout_width="wrap_content" | |
android:layout_height="wrap_content" | |
android:layout_gravity="bottom|end" | |
android:layout_margin="@dimen/fab_margin" | |
app:srcCompat="@android:drawable/ic_dialog_email" /> | |
</android.support.design.widget.CoordinatorLayout> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" | |
xmlns:app="http://schemas.android.com/apk/res-auto" | |
xmlns:tools="http://schemas.android.com/tools" | |
android:id="@+id/content_main" | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" | |
app:layout_behavior="@string/appbar_scrolling_view_behavior" | |
tools:context="com.techmobs.app.MainActivity" | |
tools:showIn="@layout/app_bar_main"> | |
<TextView | |
android:layout_width="wrap_content" | |
android:layout_height="wrap_content" | |
android:paddingBottom="@dimen/activity_vertical_margin" | |
android:paddingLeft="@dimen/activity_horizontal_margin" | |
android:paddingRight="@dimen/activity_horizontal_margin" | |
android:paddingTop="@dimen/activity_vertical_margin" | |
android:gravity="center" | |
android:textStyle="bold" | |
android:textSize="25sp" | |
android:text="You Are Not Connected To Internet : Connect To Internet And Try Again" | |
android:id="@+id/nointv" | |
android:visibility="gone"/> | |
<WebView | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" | |
android:id="@+id/webview" | |
android:layout_alignParentBottom="true" | |
android:layout_alignParentLeft="true" | |
android:layout_alignParentStart="true" /> | |
<ProgressBar | |
style="?android:attr/progressBarStyleLarge" | |
android:layout_centerHorizontal="true" | |
android:layout_height="match_parent" | |
android:layout_width="wrap_content" | |
android:layout_gravity="center_vertical|center_horizontal" | |
android:layout_alignTop="@+id/nointv" | |
android:id="@+id/prb"/> | |
</RelativeLayout> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<menu xmlns:app="http://schemas.android.com/apk/res-auto" | |
xmlns:android="http://schemas.android.com/apk/res/android"> | |
<group android:checkableBehavior="single"> | |
<item | |
android:id="@+id/nav_home" | |
android:title="Home" | |
android:icon="@drawable/home" /> | |
<item | |
android:title="Blog" | |
android:icon="@drawable/blog" | |
android:id="@+id/nav_blog" /> | |
<item | |
android:id="@+id/nav_about" | |
android:title="About" | |
android:icon="@drawable/about" /> | |
<item | |
android:id="@+id/nav_contact" | |
android:title="Contact" | |
android:icon="@drawable/contact" /> | |
</group> | |
<item android:title="More"> | |
<menu> | |
<item | |
android:id="@+id/notice" | |
android:icon="@drawable/notice" | |
android:title="Notifications" /> | |
<item | |
android:id="@+id/nav_youtube" | |
android:icon="@drawable/yt" | |
android:title="Youtube" /> | |
<item | |
android:id="@+id/nav_share" | |
android:icon="@drawable/share" | |
android:title="Share" /> | |
<item | |
android:id="@+id/nav_exit" | |
android:icon="@drawable/close" | |
android:title="Exit" /> | |
</menu> | |
</item> | |
</menu> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<menu xmlns:android="http://schemas.android.com/apk/res/android" | |
xmlns:app="http://schemas.android.com/apk/res-auto"> | |
<group android:checkableBehavior="single"/> | |
<item | |
android:id="@+id/action_whatsapp" | |
android:orderInCategory="102" | |
android:title="whatsapp" | |
android:icon="@drawable/whatsapp" | |
app:showAsAction="always" /> | |
<item | |
android:id="@+id/action_fb" | |
android:orderInCategory="102" | |
android:title="facebook" | |
android:icon="@drawable/fb" | |
app:showAsAction="always" /> | |
<item | |
android:id="@+id/action_gplus" | |
android:orderInCategory="102" | |
android:title="gplus" | |
android:icon="@drawable/gplus" | |
app:showAsAction="always" /> | |
</menu> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<resources> | |
<color name="colorPrimary">#3F51B5</color> | |
<color name="colorPrimaryDark">#202020</color> | |
<color name="colorAccent">#FF4081</color> | |
<color name="colorWhite">#ffffff</color> | |
<color name="facebook">#1636d6</color> | |
<color name="googleplus">#e60d14</color> | |
<color name="twitter">#1ccef5</color> | |
</resources> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<resources> | |
<!-- Base application theme. --> | |
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar"> | |
<!-- Customize your theme here. --> | |
<item name="colorPrimary">@color/colorPrimary</item> | |
<item name="colorPrimaryDark">@color/colorPrimaryDark</item> | |
<item name="colorAccent">@color/colorAccent</item> | |
</style> | |
<style name="AppTheme.NoActionBar"> | |
<item name="windowActionBar">false</item> | |
<item name="windowNoTitle">true</item> | |
</style> | |
<style name="AppTheme.AppBarOverlay" parent="ThemeOverlay.AppCompat.Dark.ActionBar" /> | |
<style name="AppTheme.PopupOverlay" parent="ThemeOverlay.AppCompat.Light" /> | |
</resources> |
Intent intent =newIntent(MainActivity.this,UpdateService.class)
startService(intent);
- Now watch the video and clarify if more doubts are there .
- Build your apk and then you will get your application .
Really Helpfull
ReplyDelete